This jQuery plugin provides a means for a developer to visually connect elements on their web page, in much the same way you might have seen on Yahoo Pipes. It uses Canvas in modern browsers, and Google's ExplorerCanvas script for stone-age browsers. Full transparent support for jQuery dragging is included, the API is super simple, and the compressed version of the script is just 11.6K.
You can see a demonstration here.
TweetPlumb is a great Twitter visualisation built using jsPlumb.
http://code.google.com/p/jsplumb/
Monday, April 26, 2010
DrX

DrX, the good doctor, is a small object inspector for Ruby.
DrX is for newbies and gurus alike.
Instead of focusing on the contents of your object, DrX instead focuses on its object model. As a result, DrX is most suitable for programmers wishing to understand Ruby's object model better. It's especially adept at showing you how a "magical" library works (e.g., DataMapper).
Key features:
- See everything about a Ruby object: its 'klass', 'super', 'iv_tbl', 'm_tbl'. See your singletons with your very own eyes!
- Double-click a method to launch an editor and position the cursor on the exact line where the method is defined!
Installation
At your system prompt type:gem install drx
Requirements
require 'drx'
Errors and solutions:
MissingSourceFile: no such file to load -- tk
- require 'tcltklib' (install Tcl/Tk interface for Ruby)RuntimeError: TkPackage can't find package tile
- install themed widget set provider library for TkRuntimeError: ERROR: Failed to run the 'dot' command. Make sure you have the GraphViz package installed and that its bin folder appears in your PATH.
- install rich set of graph drawing tools (GraphViz
)
Thursday, April 22, 2010
Reactive Extensions for Native JavaScript
As you may recall, Erik Meijer and his team on the Cloud Programmability Group in Building 35 have been working on the Reactive Extensions for .NET which is available on both the .NET CLR as well as Silverlight (posts). While working on the Reactive Extensions for .NET, the team also ventured into creating the same kind of functionality for native JavaScript. That means we get to use HTML and DOM events as if they were first class members instead of relegated to simple assignments. Most of the methods that are available for the .NET version are also available for the JavaScript version.
Now that we have these first class events, we can use any of the standard LINQ methods such as Select, Where, Scan, Zip and so on. We’ll cover all of those in the posts going forward. But for now, what we want to do is provide the same mouse delta dragger as we’ve had above, but this time using no “global state” and instead use composability to express our intent. With that, let’s take our mousemove and zip it together with our mouse move that skipped once so that we have an offset between our previous and our current value. Then we can take the first and second sets of our mouse events and combine them into a single object.
As you can see, we call the Skip method with a parameter of 1 which allows us to skip one instance of the mousemove firing, thus giving our offset. Then, we zip the two instances of the mousemove together to create an object which has the previous and the current mouse points. Now, what we need is a way to only fire these mouse events when the mouse button is down. Let’s look at the code required to do that.
What we did was take our mousedown observable and call the SelectMany which projects each value of an observable sequence (in this case our mousedown) to an observable sequence and flattens the resulting observable sequences into one observable sequence. Inside our SelectMany, we return our mouseMoves observable from above and we call TakeUntil passing in our mouseUp observable. Finally, much like in the Reactive Extensions for .NET, we can call subscribe to our resulting observable which allows us to set the inner HTML of our resulting div.
And there you have it, a full dragging capability using composable events in native JavaScript.
http://codebetter.com/blogs/matthew.podwysocki/archive/2010/02/16/introduction-to-the-reactive-extensions-to-javascript.aspx
$(document).ready(function() { var mouseDragMe = $("#mouseDragMe").context var mouseMove = Rx.Observable.FromHtmlEvent(mouseDragMe, "mousemove"); var mouseUp = Rx.Observable.FromHtmlEvent(mouseDragMe, "mouseup"); var mouseDown = Rx.Observable.FromHtmlEvent(mouseDragMe, "mousedown"); });
Now that we have these first class events, we can use any of the standard LINQ methods such as Select, Where, Scan, Zip and so on. We’ll cover all of those in the posts going forward. But for now, what we want to do is provide the same mouse delta dragger as we’ve had above, but this time using no “global state” and instead use composability to express our intent. With that, let’s take our mousemove and zip it together with our mouse move that skipped once so that we have an offset between our previous and our current value. Then we can take the first and second sets of our mouse events and combine them into a single object.
var mouseMoves = mouseMove .Skip(1) .Zip(mouseMove, function(left, right) { return { x1 : left.clientX, y1 : left.clientY, x2 : right.clientX, y2 : right.clientY }; });
var mouseDrags = mouseDown.SelectMany(function(md) { return mouseMoves.TakeUntil(mouseUp); });
mouseDrags.Subscribe(function(mouseEvents) { $("#results").html( "Old (X: " + mouseEvents.x1 + " Y: " + mouseEvents.y1 + ") " + "New (X: " + mouseEvents.x2 + " Y: " + mouseEvents.y2 + ")"); });
http://codebetter.com/blogs/matthew.podwysocki/archive/2010/02/16/introduction-to-the-reactive-extensions-to-javascript.aspx
Wednesday, April 21, 2010
Greenhouse
Greenhouse: Graphical Interface Builder for building blazing fast native-style applications using SproutCore.
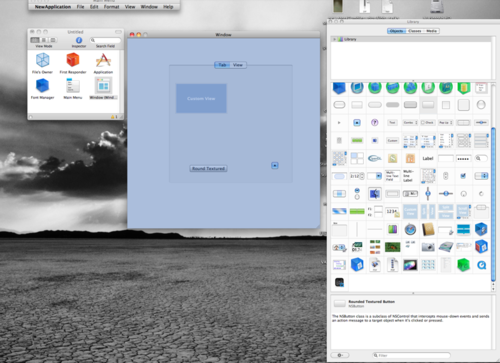
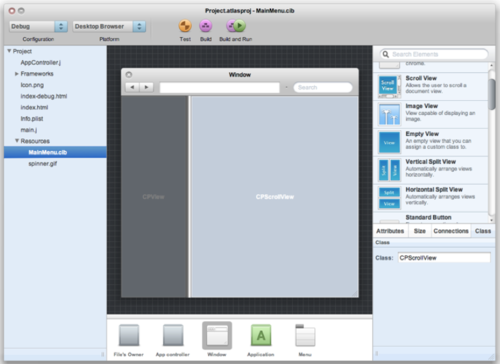
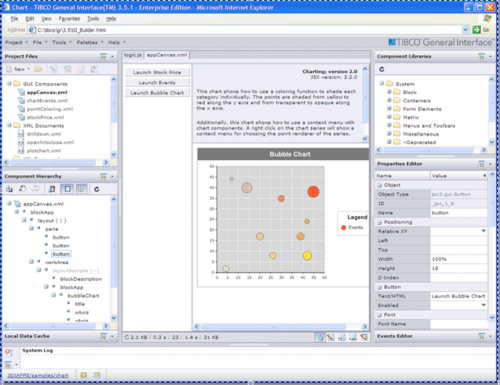
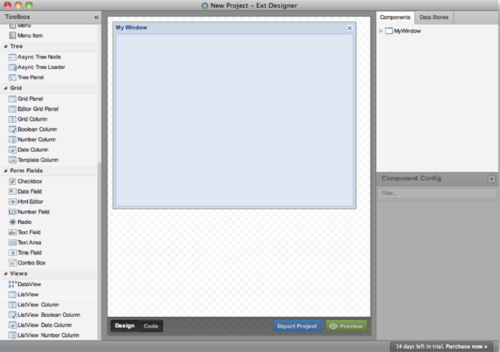
So these are good, but they all have the same problem.
If we are to build beautiful web applications, we can’t do it with a cluttered workspace. We need a workspace for creativity, but these interfaces are cluttered with controls and only give you a small window to see your actual content!
So what can we innovate this space? How do we turn these cramped UIs in to a place where we can create some awesome web applications? Here are the steps that we took:
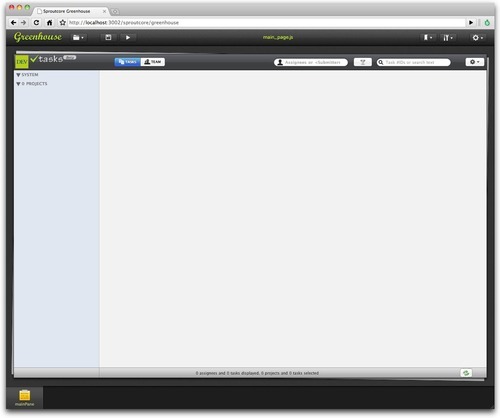

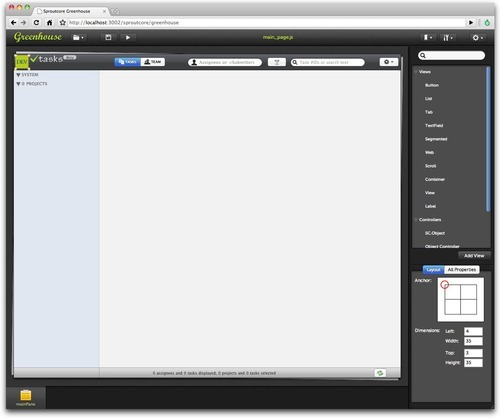
In this way, we maximize the workspace. We move as much as possible out of the way so you can focus on your content. This is also a true WYSIWYG editor.
We are all busy people. Most of us don’t have designers that can build custom mockups of views to show us what our UI will look like. Also, these designs change so much that is a major feat to keep up to date if you do have a designer. It is a hugh waste of time. So what you are forced to do is actually not have your custom views styled in your UI builder and it looks like this:

The SproutCore team decided the best way to solve this was to leverage the power of JavaScript. Rather than load a data file, Greenhouse actually loads your entire application in an iframe. Your actual application is loaded in a ‘suspended’ design state so that you can actually see your custom views as you have built them. They will update and look exactly like they would in the code.
This is an amazing time saver for you. It can help you actually build amazing user interfaces. This is what it looks like with a large application with a lot of custom views. It looks exactly like it does in real life as if you were using it!
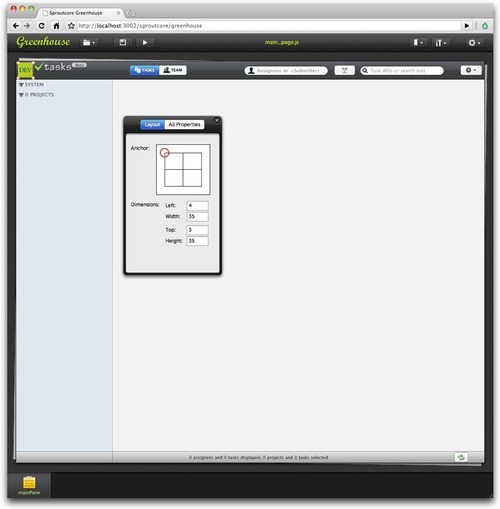
Oh, and one more thing: Greenhouse’s file format is actually Javascript built off of the 1.0 API. If you have a Sproutcore App that is built with SC.Pages, your pages will automatically load into Greenhouse will little to no effort.
We on the SproutCore Team believe that a UI designer is a fundamental tool for any framework that is worth it’s salt. So we have decided to build Greenhouse into SproutCore - entirely free. We want to give this away because its the right thing to do.
git clone git://github.com/sproutit/sproutcore-abbot.git abbot
cd abbot
mkdir frameworks
cd frameworks
git clone git://github.com/sproutit/sproutcore.git
cd ../..
And then from your Sproutcore project directory:
<path_to>/abbot/bin/sc-server
Now all you have to do is open: http://localhost:4020/sproutcore/greenhouse you should get the App Viewer:
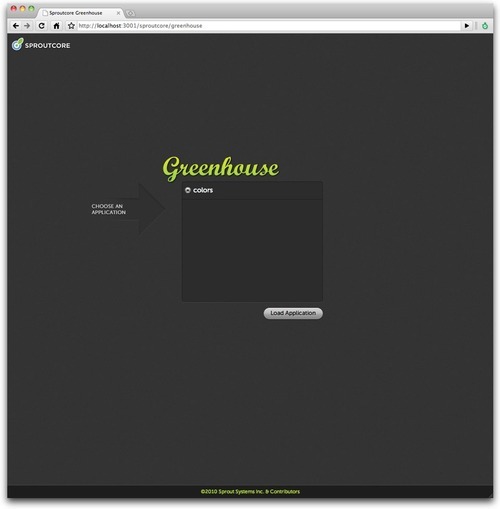
Note: If you use a custom build of SproutCore (something in frameworks/sproutcore), you will need to rebase to the Top-Of-Tree (TOT) to make sure you have all the code to run your application in Greenhouse.
http://blog.sproutcore.com/post/535950751/introducing-greenhouse
So What, Everyone Has a Interface Builder…
While this may be true, no one has really innovated in this space for 20 years. They all seem to look the same. Here is a small sample of them:Interface Builder
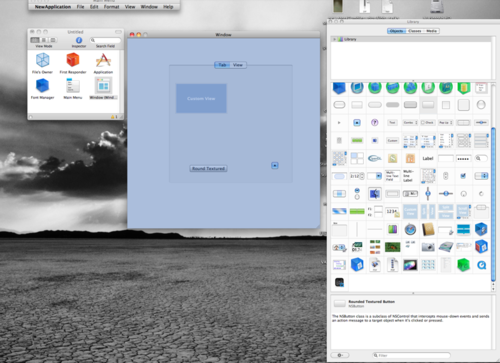
Atlas
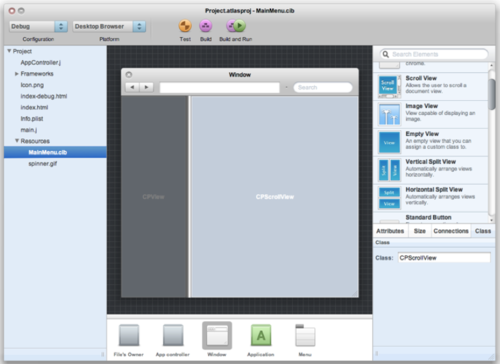
TIBCO Interface
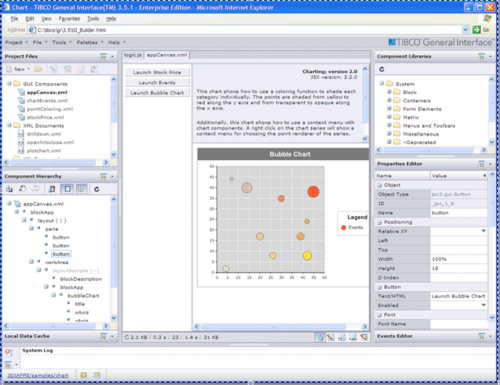
Ext-JS Builder
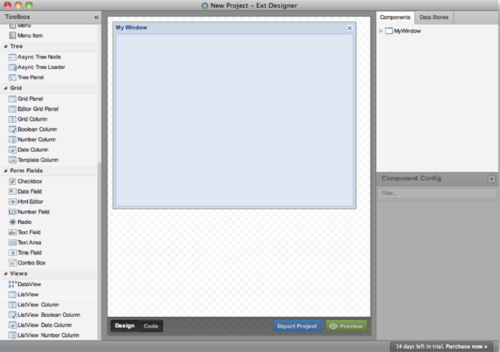
So these are good, but they all have the same problem.
If we are to build beautiful web applications, we can’t do it with a cluttered workspace. We need a workspace for creativity, but these interfaces are cluttered with controls and only give you a small window to see your actual content!
So what can we innovate this space? How do we turn these cramped UIs in to a place where we can create some awesome web applications? Here are the steps that we took:
Clean Up the UI
First, we cleaned up the interface. We move the list of components and inspectors to picker panes that can be torn off and docked in the traditional interface builders.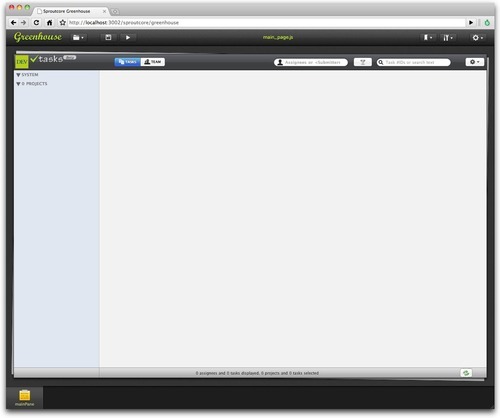

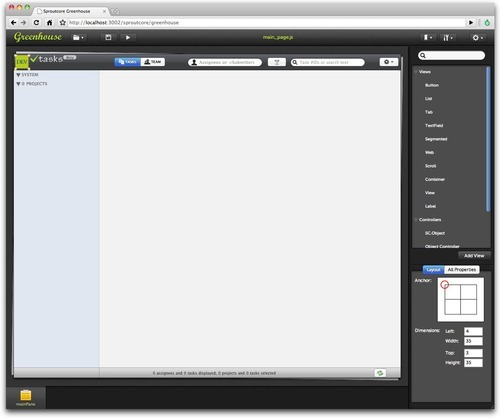
In this way, we maximize the workspace. We move as much as possible out of the way so you can focus on your content. This is also a true WYSIWYG editor.
Solving the Blue Box Problem
Most production apps are built from custom views developed for the app. The problem is most interface builders can’t show you these custom views so they draw a blue box and leave it to your imagination.We are all busy people. Most of us don’t have designers that can build custom mockups of views to show us what our UI will look like. Also, these designs change so much that is a major feat to keep up to date if you do have a designer. It is a hugh waste of time. So what you are forced to do is actually not have your custom views styled in your UI builder and it looks like this:

The SproutCore team decided the best way to solve this was to leverage the power of JavaScript. Rather than load a data file, Greenhouse actually loads your entire application in an iframe. Your actual application is loaded in a ‘suspended’ design state so that you can actually see your custom views as you have built them. They will update and look exactly like they would in the code.
This is an amazing time saver for you. It can help you actually build amazing user interfaces. This is what it looks like with a large application with a lot of custom views. It looks exactly like it does in real life as if you were using it!
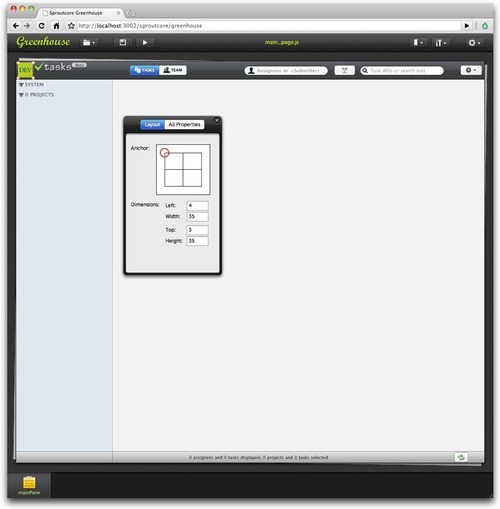
Oh, and one more thing: Greenhouse’s file format is actually Javascript built off of the 1.0 API. If you have a Sproutcore App that is built with SC.Pages, your pages will automatically load into Greenhouse will little to no effort.
OK, That’s nice, but what is the catch?
Well, most interface builders cost lots of money. Like $100, $200 and even more. Some will even charge you for their beta releases.We on the SproutCore Team believe that a UI designer is a fundamental tool for any framework that is worth it’s salt. So we have decided to build Greenhouse into SproutCore - entirely free. We want to give this away because its the right thing to do.
Cool! How do I get it?
Well, that’s the easy part. Greenhouse is currently built directly into Sproutcore with some really easy steps to get it:git clone git://github.com/sproutit/sproutcore-abbot.git abbot
cd abbot
mkdir frameworks
cd frameworks
git clone git://github.com/sproutit/sproutcore.git
cd ../..
And then from your Sproutcore project directory:
<path_to>/abbot/bin/sc-server
Now all you have to do is open: http://localhost:4020/sproutcore/greenhouse you should get the App Viewer:
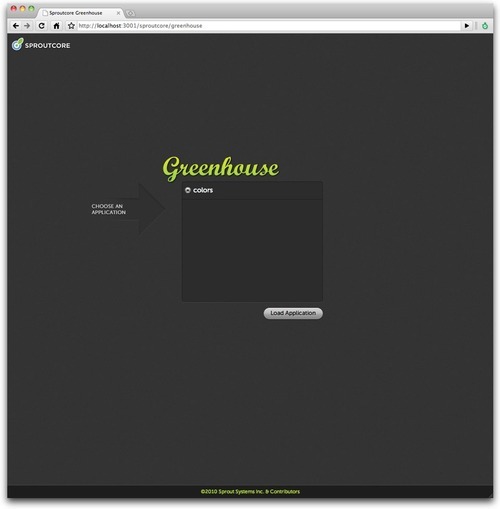
Note: If you use a custom build of SproutCore (something in frameworks/sproutcore), you will need to rebase to the Top-Of-Tree (TOT) to make sure you have all the code to run your application in Greenhouse.
http://blog.sproutcore.com/post/535950751/introducing-greenhouse
Monday, April 19, 2010
Non-blocking ActiveRecord & Rails

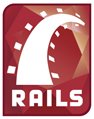
Dissecting Ruby MySQL drivers
The native mysql gem many of us use in production was designed to expose a blocking API: you issue a SQL query, and the library blocks until the server returns a response. So far so good, but unfortunately it also introduces a nasty side effect. Because it blocks inside of the native code (inside mysql_real_query() C function), the entire Ruby VM is frozen while we wait for the response. So, if you query happens to have taken several seconds, it means that no other block, fiber, or thread will be executed by the Ruby VM. Ever wondered why your threaded Mongrel server never really flexed its threaded muscle? Well, now you know.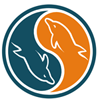
http://www.igvita.com/2010/04/15/non-blocking-activerecord-rails/?utm_source=feedburner&utm_campaign=Feed%3A+igvita+%28igvita.com%29&utm_content=feed
Sunday, April 18, 2010
Making AJAX Applications Crawlable
Ajax: The Definitive Guide
This document outlines the steps that are necessary in order to make your AJAX application crawlable. Once you have fully understood each of these steps, it should not take you very long to actually make your application crawlable! However, you do need to understand each of the steps involved, so we recommend reading this guide in its entirety.
Briefly, the solution works as follows: the crawler finds a pretty AJAX URL (that is, a URL containing a

http://code.google.com/intl/sv-SE/web/ajaxcrawling/docs/getting-started.html
This document outlines the steps that are necessary in order to make your AJAX application crawlable. Once you have fully understood each of these steps, it should not take you very long to actually make your application crawlable! However, you do need to understand each of the steps involved, so we recommend reading this guide in its entirety.
Briefly, the solution works as follows: the crawler finds a pretty AJAX URL (that is, a URL containing a
#!
hash fragment). It then requests the content for this URL from your server in a slightly modified form. Your web server returns the content in the form of an HTML snapshot, which is then processed by the crawler. The search results will show the original URL. 
http://code.google.com/intl/sv-SE/web/ajaxcrawling/docs/getting-started.html
jStorage - store data locally with JavaScript
jStorage is a simple wrapper plugin for Prototype, MooTools and jQuery to cache data on browser side.
jStorage was first developed under the name of DOMCached but since a lot of features were dropped to make it simpler (like the support for namespaces and such) it was renamed. DOMCached had separate files for working with Prototype and jQuery but jStorage can handle both in one go.
http://www.jstorage.info/
Rails HTTP Status Code to Symbol Mapping
The symbol used is an underscored version of the status message with no spaces. A quick Ruby script yields the following mapping:
http://www.codyfauser.com/2008/7/4/rails-http-status-code-to-symbol-mapping
Status Code | Status Message | Symbol |
---|---|---|
1xx Informational | ||
100 | Continue | :continue |
101 | Switching Protocols | :switching_protocols |
102 | Processing | :processing |
2xx Success | ||
200 | OK | :ok |
201 | Created | :created |
202 | Accepted | :accepted |
203 | Non-Authoritative Information | :non_authoritative_information |
204 | No Content | :no_content |
205 | Reset Content | :reset_content |
206 | Partial Content | :partial_content |
207 | Multi-Status | :multi_status |
226 | IM Used | :im_used |
3xx Redirection | ||
300 | Multiple Choices | :multiple_choices |
301 | Moved Permanently | :moved_permanently |
302 | Found | :found |
303 | See Other | :see_other |
304 | Not Modified | :not_modified |
305 | Use Proxy | :use_proxy |
307 | Temporary Redirect | :temporary_redirect |
4xx Client Error | ||
400 | Bad Request | :bad_request |
401 | Unauthorized | :unauthorized |
402 | Payment Required | :payment_required |
403 | Forbidden | :forbidden |
404 | Not Found | :not_found |
405 | Method Not Allowed | :method_not_allowed |
406 | Not Acceptable | :not_acceptable |
407 | Proxy Authentication Required | :proxy_authentication_required |
408 | Request Timeout | :request_timeout |
409 | Conflict | :conflict |
410 | Gone | :gone |
411 | Length Required | :length_required |
412 | Precondition Failed | :precondition_failed |
413 | Request Entity Too Large | :request_entity_too_large |
414 | Request-URI Too Long | :request_uri_too_long |
415 | Unsupported Media Type | :unsupported_media_type |
416 | Requested Range Not Satisfiable | :requested_range_not_satisfiable |
417 | Expectation Failed | :expectation_failed |
422 | Unprocessable Entity | :unprocessable_entity |
423 | Locked | :locked |
424 | Failed Dependency | :failed_dependency |
426 | Upgrade Required | :upgrade_required |
5xx Server Error | ||
500 | Internal Server Error | :internal_server_error |
501 | Not Implemented | :not_implemented |
502 | Bad Gateway | :bad_gateway |
503 | Service Unavailable | :service_unavailable |
504 | Gateway Timeout | :gateway_timeout |
505 | HTTP Version Not Supported | :http_version_not_supported |
507 | Insufficient Storage | :insufficient_storage |
510 | Not Extended | :not_extended |
http://www.codyfauser.com/2008/7/4/rails-http-status-code-to-symbol-mapping
Thursday, April 1, 2010
Postgres 9.1 - Release Theme
Following a great deal of discussion, I'm pleased to announce that the PostgreSQL Core team has decided that the major theme for the 9.1 release, due in 2011, will be 'NoSQL'.
There is a growing trend towards NoSQL databases, with major sites like Twitter and Facebook utilising them extensively. NoSQL databases often include multi-master replication, clustering and failover features that have long been requested in PostgresSQL, but have been extremely difficult to implement with SQL which has prevented us from advancing Postgree in the way that we'd like.
To address this, the intention is to remove SQL support from Postgres, and replace it with a language called 'QUEL'. This will provide us with the flexibility we need to implement the features of modern NoSQL databases. With no SQL support there will obviously be some differences in the query syntax that must be used to access your data. For example, the query:
select (e.salary/ (e.age - 18)) as comp from employee as e where e.name = "Jones"
would be rewritten as:
range of e is employee retrieve (comp = e.salary/ (e.age - 18)) where e.name = "Jones"
Aggregate syntax in QUEL is particularly powerful. For example, the query:
range of e is employee
We will be producing a comprehensive guide to the QUEL syntax to aid with application migration. We appreciate the difficulty that this change may cause some users, but feel we must embrace the NoSQL philosophy in order to remain "The world's most advanced Open Source database"
"There's no question that, at 21 years old, the SQL standard is past its prime," said core developer and standards expert Peter Eisentraut. "It's time for us to switch to something fresher. I personally would have preferred XSLT, but QUEL is almost as good."
Project committer Heikki Linnakangas added: "By replacing SQL with QUEL not only will will be able to add new features to Postgres that were previously too difficult, but we'll also increase user loyalty as it'll be much harder for them to change to a different, SQL-based database. That'll be pretty cool."
You may also notice that without SQL, the project name is somewhat misleading. To address that, the project name will be changed to 'PostgreQUEL' with the 9.1 release. We expect this will also put an end to the periodic debates on changing the project name.
Dave Page
On behalf of the PostgreSQL Core Team
http://archives.postgresql.org/pgsql-hackers/2010-04/msg00003.php
There is a growing trend towards NoSQL databases, with major sites like Twitter and Facebook utilising them extensively. NoSQL databases often include multi-master replication, clustering and failover features that have long been requested in PostgresSQL, but have been extremely difficult to implement with SQL which has prevented us from advancing Postgree in the way that we'd like.
To address this, the intention is to remove SQL support from Postgres, and replace it with a language called 'QUEL'. This will provide us with the flexibility we need to implement the features of modern NoSQL databases. With no SQL support there will obviously be some differences in the query syntax that must be used to access your data. For example, the query:
select (e.salary/ (e.age - 18)) as comp from employee as e where e.name = "Jones"
would be rewritten as:
range of e is employee retrieve (comp = e.salary/ (e.age - 18)) where e.name = "Jones"
Aggregate syntax in QUEL is particularly powerful. For example, the query:
select dept,may be written as:
avg(salary) as avg_salary,
sum(salary) as tot_salary
from
employees
group by
dept
range of e is employee
retrieve (e.dept,Note that the grouped column can be specified for each individual aggregate.
avg_salary = avg(e.salary by e.dept),
tot_salary = sum(e.salary by e.dept)
)
We will be producing a comprehensive guide to the QUEL syntax to aid with application migration. We appreciate the difficulty that this change may cause some users, but feel we must embrace the NoSQL philosophy in order to remain "The world's most advanced Open Source database"
"There's no question that, at 21 years old, the SQL standard is past its prime," said core developer and standards expert Peter Eisentraut. "It's time for us to switch to something fresher. I personally would have preferred XSLT, but QUEL is almost as good."
Project committer Heikki Linnakangas added: "By replacing SQL with QUEL not only will will be able to add new features to Postgres that were previously too difficult, but we'll also increase user loyalty as it'll be much harder for them to change to a different, SQL-based database. That'll be pretty cool."
You may also notice that without SQL, the project name is somewhat misleading. To address that, the project name will be changed to 'PostgreQUEL' with the 9.1 release. We expect this will also put an end to the periodic debates on changing the project name.
Dave Page
On behalf of the PostgreSQL Core Team
http://archives.postgresql.org/pgsql-hackers/2010-04/msg00003.php
Subscribe to:
Posts (Atom)
Bookmarks
Generators
- .NET Buttons
- 3D-box maker
- A CSS sticky footer
- A web-based graphics effects generator
- Activity indicators
- Ajax loader
- ASCII art generator
- Attack Ad Generator
- Badge shape creation
- Binary File to Base64 Encoder / Translator
- Browsershots makes screenshots of your web design in different browsers
- Button generator
- Buttonator 2.0
- Color Palette
- Color schemer
- Color Themes
- Colorsuckr: Create color schemes based on photos for use in your artwork & designs
- Create DOM Statements
- CSS Organizer
- CSS Sprite Generator
- CSS Sprites
- CSS Type Set
- Digital Post It Note Generator
- Easily create web forms and fillable PDF documents to embed on your websites
- egoSurf
- Favicon Editor
- Favicon generator
- Flash website generator
- Flip Title
- Flipping characters with UNICODE
- Form Builder
- Free Footer online tools for webmasters and bloggers.
- Free templates
- FreshGenerator
- Genfavicon
- hCalendar Creator
- HTML form builder
- HTML to Javascript DOM converter
- Image Mosaic Generator
- Image reflection generator
- img2json
- JSON Visualization
- Login form design patterns
- Logo creator
- Lorem Ipsum Generator
- LovelyCharts
- Markup Generator
- Mockup Generator
- Online Background Generators
- PatternTap
- Pixenate Photo Editor
- Preloaders
- Printable world map
- punypng
- Regular Expressions
- RoundedCornr
- SingleFunction
- Spam proof
- Stripe designer
- Stripe generator 2.0
- Tabs generator
- Tartan Maker. The new trendsetting application for cool designers
- Test Everithing
- Text 2 PNG
- The Color Wizard 3.0
- tinyarro.ws: Shortest URLs on Earth
- Web 2.0 Badges
- Web UI Development
- Website Ribbon
- wwwsqldesigner
- Xenocode Browser Sandbox - Run any browser from the web
- XHTML/CSS Markup generator
Library
- 12 Steps to MooTools Mastery
- AJAX APIs Playground
- Best Tech Videos
- CSS Tricks
- FileFormat.info
- Grafpedia
- IT Ebooks :: Videos
- Learning Dojo
- Linux Software Repositories
- NET Books
- PDFCHM
- Rails Engines
- Rails Illustrated
- Rails Metal: a micro-framework with the power of Rails: \m/
- Rails Podcast
- Rails Screencasts
- RegExLib
- Ruby On Rails Security Guide
- Ruby-GNOME2 Project Website
- Rubyology
- RubyPlus Video
- Scaling Rails
- Scripteka
- This Week in Django
- WebAppers